版本
springboot 2.1.3
swagger2 2.9.2
仓库案例:springboot_swagger
引入依赖
1 2 3 4 5 6 7 8 9 10 11 12
| <dependency> <groupId>io.springfox</groupId> <artifactId>springfox-swagger2</artifactId> <version>2.9.2</version> </dependency>
<dependency> <groupId>io.springfox</groupId> <artifactId>springfox-swagger-ui</artifactId> <version>2.9.2</version> </dependency>
|
新增配置类swagger.java
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19
| package com.karoy.springboot.config;
import io.swagger.annotations.ApiOperation; import org.springframework.context.annotation.Bean; import org.springframework.context.annotation.Configuration; import springfox.documentation.builders.RequestHandlerSelectors; import springfox.documentation.spi.DocumentationType; import springfox.documentation.spring.web.plugins.Docket; import springfox.documentation.swagger2.annotations.EnableSwagger2;
@Configuration @EnableSwagger2 public class swagger { @Bean public Docket swaggerSpringMvcPlugin() { return new Docket(DocumentationType.SWAGGER_2).select().apis(RequestHandlerSelectors.withMethodAnnotation(ApiOperation.class)).build(); } }
|
api方法增加注解
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28
| package com.karoy.springboot.action;
import com.karoy.springboot.common.ApiResult; import io.swagger.annotations.Api; import io.swagger.annotations.ApiOperation; import io.swagger.annotations.ApiResponse; import io.swagger.annotations.ApiResponses; import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.bind.annotation.RequestParam; import org.springframework.web.bind.annotation.RestController;
@Api(description = "HELLO接口") @RestController public class HelloController { @ApiOperation(value = "欢迎" , notes="欢迎") @ApiResponses({ @ApiResponse(code = 200, message = "OK", response = ApiResult.class), @ApiResponse(code = 204, message = "No Content", response = ApiResult.class), @ApiResponse(code = 401, message = "Unauthorized", response = ApiResult.class), @ApiResponse(code = 403, message = "Forbidden", response = ApiResult.class), @ApiResponse(code = 404, message = "Not Found", response = ApiResult.class) }) @RequestMapping("/hello") public String hello(@RequestParam("name") String name) { return "Hello "+name; } }
|
ApiResult
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59
| package com.karoy.springboot.common;
import io.swagger.annotations.ApiModel; import io.swagger.annotations.ApiModelProperty;
import java.io.Serializable;
@ApiModel(value = "ApiResult",description = "api接口通用返回对象") public class ApiResult implements Serializable {
@ApiModelProperty(value = "返回码",dataType = "int") private int code;
@ApiModelProperty(value = "错误信息",dataType = "String") private String message;
@ApiModelProperty(value = "响应数据",dataType = "Object") private Object data;
public final int getCode() { return this.code; }
public final void setCode(int code) { this.code = code; }
public final String getMessage() { return this.message; }
public final void setMessage( String message) { this.message = message; }
public final Object getData() { return this.data; }
public final void setData(Object data) { this.data = data; }
public ApiResult(int code, String message, Object data) { super(); this.code = code; this.message = message; this.data = data; } }
|
访问swagger-ui.html
应用启动后访问 http://localhost:8080/swagger-ui.html
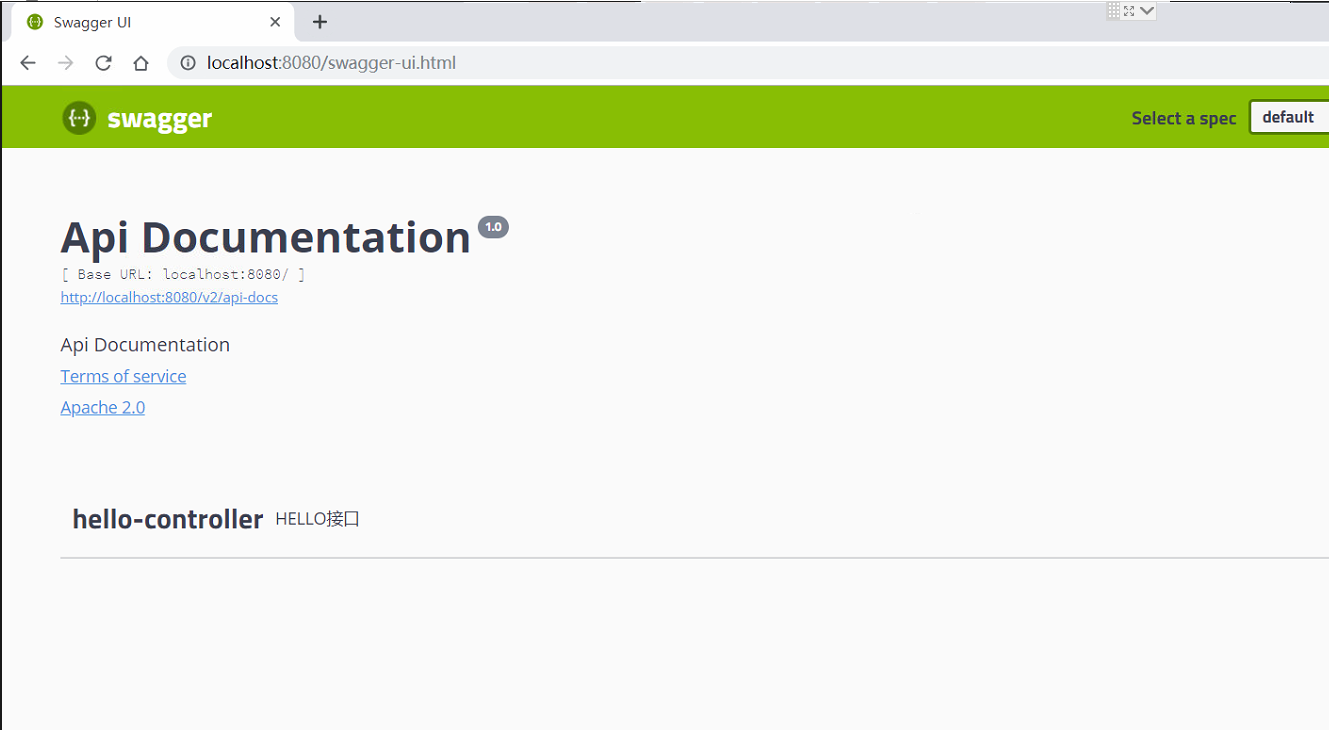
点击hello-controller
可以看到详细接口定义
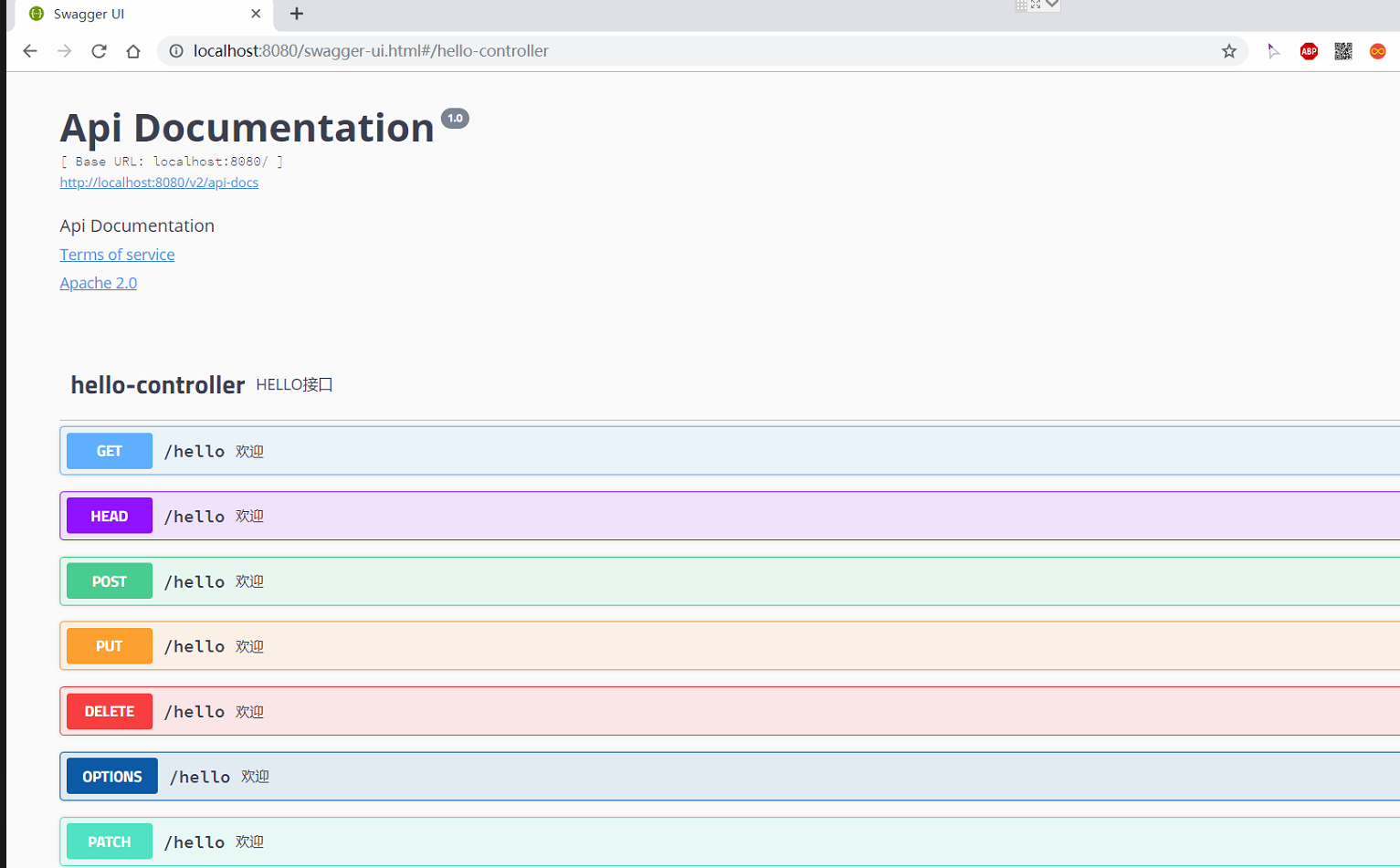
点击具体接口可以看到具体参数
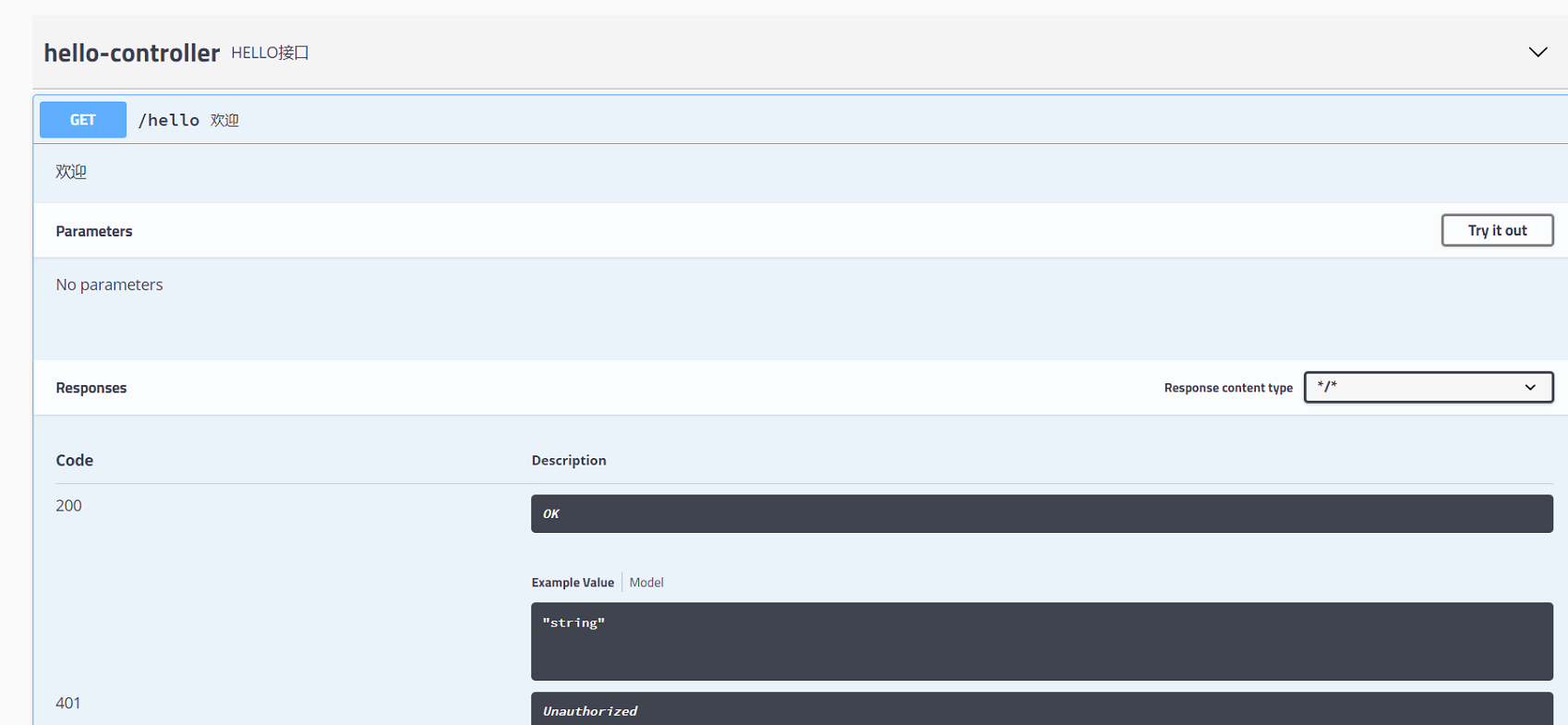
点击Try out
并execute
可以看到返回结果
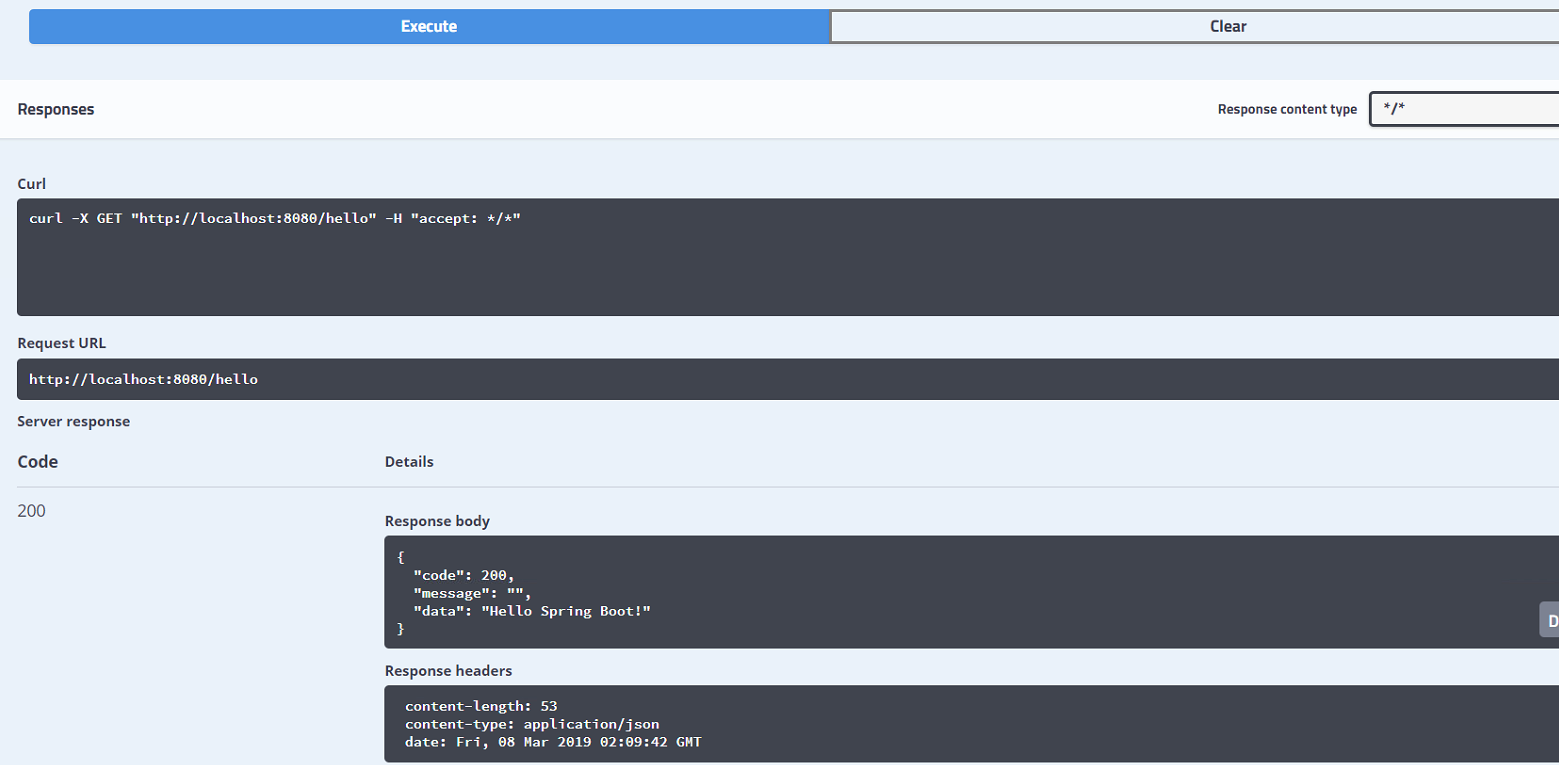
常用注解
@Api:用在类上,说明该类的作用
@ApiOperation:用在方法上,说明方法的作用
@ApiImplicitParams:用在方法上包含一组参数说明
@ApiImplicitParam:用在@ApiImplicitParams注解中,指定一个请求参数的各个方面
paramType:参数放在哪个地方
header-->请求参数的获取:@RequestHeader
query-->请求参数的获取:@RequestParam
path(用于restful接口)-->请求参数的获取:@PathVariable
body
form
name:参数名
dataType:参数类型
required:参数是否必须传
value:参数的意思
defaultValue:参数的默认值
@ApiResponses:用于表示一组响应
@ApiResponse:用在@ApiResponses中,一般用于表达一个错误的响应信息
code:数字,例如400
message:信息,例如"请求参数没填好"
response:抛出异常的类
@ApiModel:描述一个Model的信息(这种一般用在post创建的时候,使用@RequestBody这样的场景,请求参数无法使用@ApiImplicitParam注解进行描述的时候)
@ApiModelProperty:描述一个model的属性
以上这些就是最常用的几个注解了。
结束